DialogEx
Shows a dialog that can be used to specify script parameters. Python only.
Syntax
DialogEx(dialogSpecString)
Parameters
Parameter |
Type |
Description |
---|---|---|
dialogSpecString |
string |
Dialog specification saved as JSON string. See example below. |
Return
Returns JSON string representing a Python dictionary dlgRes
with two fields:
dlgRes["dialogResult"]
is a boolean value (True
if user pressed OK in dialog,False
if user pressed Cancel in dialog).dlgRes["parameters"]
is a list of dialog parameters with parameter values specified in the dialog.
Dialog parameter is a Python dictionary par
with the following fields:
par["Type"]
- a string specifying parameter type. Should be"Number"
,"Integer"
,"String"
,"Boolean"
or"Choice"
.par["Name"]
- a string specifying parameter name in the dialog.par["Description"]
- a string specifying parameter description in the dialog (shown in the lower panel of the dialog when a parameter is selected).par["Value"]
- parameter value. Should be:float
for"Number"
parameter typeint
for"Integer"
parameter typestr
for"String"
parameter typebool
for"Boolean"
parameter typeint
for"Choice"
parameter type
par["List"]
- a list of choices if parameter type is"Choice"
.
Examples
Python
import nex
import json
def MakePar(typeString, nameString, descString, value, list=[]):
""" Makes parameter dict used in nex.DialogEx. """
return {"Type": typeString, "Name": nameString, "Description": descString, "Value": value, "List": list }
p1 = MakePar("Number", "float par name", "float par description", 3.14 )
p2 = MakePar("String", "string par name", "string par description", "abc" )
p3 = MakePar("Integer", "integer par", "some description", 7 )
p4 = MakePar("Boolean", "bool par", "Bool description", False )
p5 = MakePar("Choice", "choice par", "Trying choice type", 1, ["one", "two", "three" ] )
doc = nex.GetActiveDocument()
p6 = MakePar("Choice", "Select Neuron", "Select Neuron from the list of neurons", 1, doc.NeuronNames() )
pars = [p1, p2, p3, p4, p5, p6]
dlgPars = {"DialogDescription": "Some dialog description", "DialogTitle": "My Script Parameters", "Parameters": pars}
res = nex.DialogEx(json.dumps(dlgPars))
dialogResultDict = json.loads(res)
print(dialogResultDict)
The following dialog will be shown:
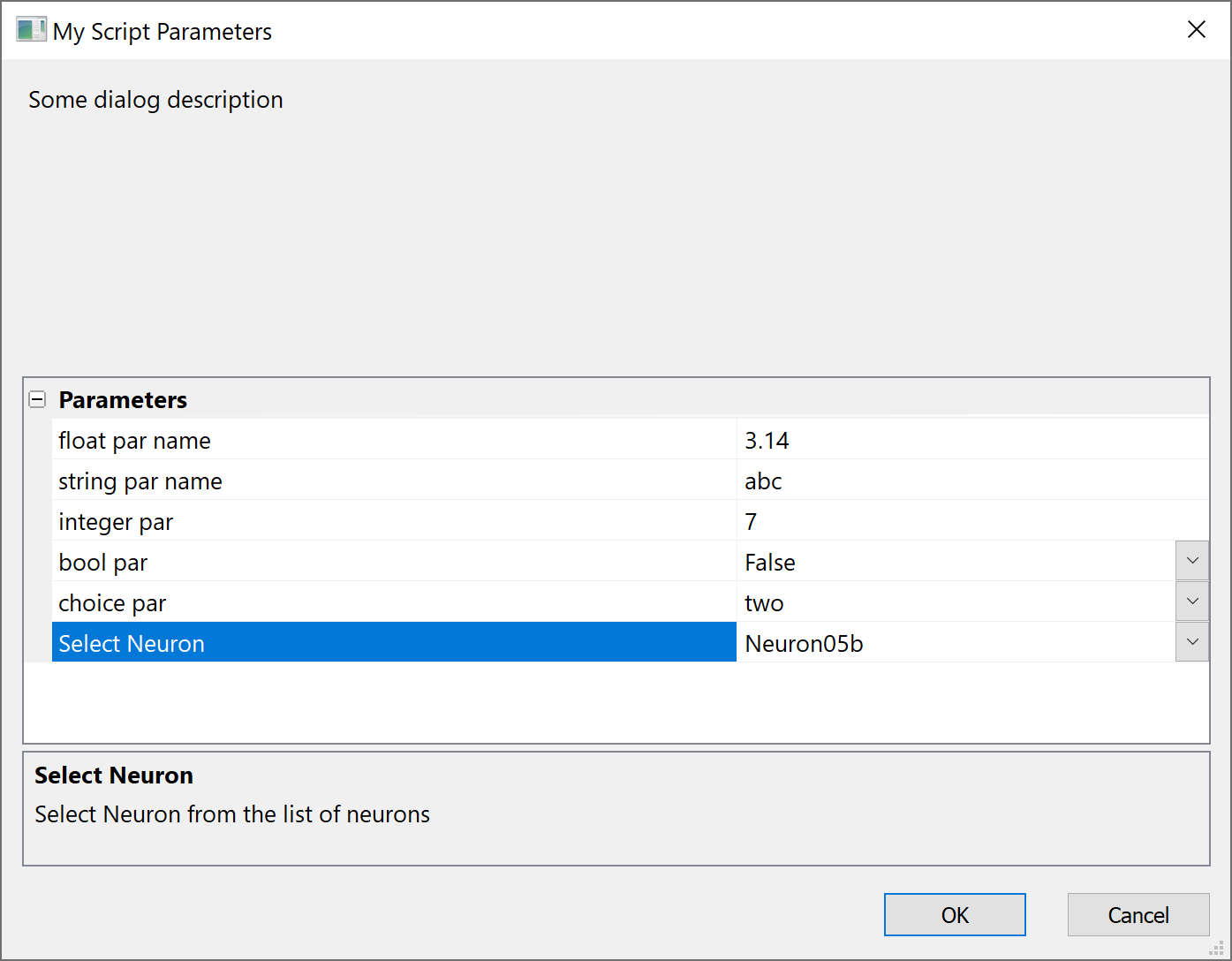